


My C# library to read, write, and create maps.

Feature List:





The content of the archive:



Library documentation 

Cs2dMapLib 

Classes 

1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
Class: Map //Class for storing map data Constructors: public Map(string Path) //Open a map public Map(int SizeX, int SizeY) //Create new map Methods: public void Save(string Path) //Save a map Properties: public string HeaderStart; //It is not recommended to change public bool BackScroling; //Background scrolling public int Check_ms; //Unknown public int AuthorId; //Author USGN public int DaylightTime; //Daylight Time public string AuthorNick; //Author Nickname public string Code; //It is not recommended to change public string TilesName; //Tileset Name public string BackName; //Background file name public XY BackScrollSpeed; //Background move speed public TColor BackColor; //Background color public string HeaderEnd; //It is not recommended to change public TileSet TSet; //Tileset info public TileMap TMap; //Tilemap info public List<Entity> Entites; //Entity list
1
2
3
4
5
6
7
8
9
10
2
3
4
5
6
7
8
9
10
Class: TileMap //Class for storing tile map data Constructor: public TileMap(int SizeX, int SizeY) //Create a tiled map of size Method: BlendCheck() //Check if this map uses textures blend Properties: public int SizeX { get; private set; } //Size map X public int SizeY { get; private set; } //Size map Y public TileInfo[,] Tiles; //Tile massive. Initialized when calling the constructor. ...= new TileInfo[SizeX, SizeY]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
Class: TileInfo //Class for storing the data tile Constructor: public TileInfo() //Create tile info Methods: public void SetBlend(BlentType Type, BlendRotate Rotate) //Set blend tile and reset color tile public void SetColor(byte R, byte G, byte B) //Set color tile and reset blend tile public void ClearModify() //Clear blend and color tile public void ClearAllModify() //Clear blend, color, rotation and brightness tile public bool BlendCheck() //Check for the presence of tile modifications public byte GetModByte() //Return the type of tiles modification in the form of Byte public byte GetBlendByte() //Return data on the tiles blend in the form of Byte public byte[] GetColorByteMassive() //Return an array of bytes to the cell color. {TileColor.R, TileColor.G, TileColor.B, Overlay} Properties: public byte Tile; //Tile id public Rotation TRotation; //Tile rotation public Brightness TBrightness; //Tile brightness public ModifyType ModType { get; private set; } //Tile modification type public BlentType BType { get; private set; } //Tile blend type public BlendRotate BRotate { get; private set; } //Tile blend rotate public TColor TileColor { get; private set; } //Tile rgb color public byte Overlay; //Unknown
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
Class: Entity //Class for storing the data entity Constructors: public Entity(string Name, EntityType Type, XY Position, string Trigger, int[] Ints, string[] Strs) //Create new entity public Entity(string Name, EntityType Type, XY Position, string Trigger) //Create new entity public Entity(string Name, EntityType Type, XY Position) //Create new entity public Entity(EntityType Type, XY Position, string Trigger) //Create new entity public Entity(EntityType Type, XY Position) //Create new entity Method: private void CreateMassives() //Used in the constructors. Creates arrays with entity parameters Properties: public string Name; //Entity name public EntityType Type; //Entity type public XY Position; //Entity position public string Trigger; //Entity trigger public int[] Ints; //If an entity has some parameters such as integer, they are stored in the array. For example, rotation, radiobutton index or id public string[] Strs; //If the object has any parameters such as integer, they are stored in the array. For example, the path or line with a message
1
2
3
4
5
6
7
8
9
10
2
3
4
5
6
7
8
9
10
Class: TColor //Class storage rgb color Constructor: public TColor(byte R, byte G, byte B) //Create rgb color Method: public byte[] GetColorBytes() //Return an array of bytes with color Properties: public byte R; //Red public byte G; //Green public byte B; //Blue
1
2
3
4
5
6
7
8
9
2
3
4
5
6
7
8
9
Class: XY //Class storage 2d coordinates Constructor: public XY(int X, int Y) //Create 2d coordinates Method: public int[] GetArray() //Return an array of integer with 2d coordinates Properties: public int X; //X position public int Y; //Y position
1
2
3
4
5
6
7
8
9
2
3
4
5
6
7
8
9
Class: TileSet //Class storage tileset. ...gfx/tiles/[name].inf Constructors: public TileSet(byte Count) //Create tileset of a predetermined number of rings public TileSet(string TilesetInfoFile) //Open the tileset file on the specified path Method: public void Save(string Path) //Save tileset along a predetermined path Propertie: public TileMode[] Tile; //An array of parameters tileset tiles
Enums 

1
2
3
4
5
6
7
2
3
4
5
6
7
public enum Rotation { 	Up = 0, 	Right = 1, 	Down = 2, 	Left = 3 };
1
2
3
4
5
6
7
8
9
10
11
12
13
2
3
4
5
6
7
8
9
10
11
12
13
public enum Brightness { 	b_10 = 4, 	b_20 = 8, 	b_30 = 12, 	b_40 = 16, 	b_50 = 20, 	b_60 = 24, 	b_70 = 28, 	b_80 = 32, 	b_90 = 36, 	b_100 = 0 }
1
2
3
4
5
6
2
3
4
5
6
public enum ModifyType { 	None = 0, 	Blend = 64, 	Color = 128 };
1
2
3
4
5
6
7
8
2
3
4
5
6
7
8
public enum BlendType { 	LinearS = 0, 	LinearH = 8, 	DirtS = 16, 	DirtH = 24, 	Grass = 32 };
1
2
3
4
5
6
7
8
9
10
11
2
3
4
5
6
7
8
9
10
11
public enum BlendRotate { 	r_0 = 0, 	r_45 = 1, 	r_90 = 2, 	r_135 = 3, 	r_180 = 4, 	r_225 = 5, 	r_270 = 6, 	r_315 = 7 };
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
public enum TileMode { 	FloorSoundless = 0, 	Wall = 1, 	Obstacle = 2, 	WallNoShadow = 3, 	ObstacleNoShadow = 4, 	FloorDirt = 10, 	FloorSnow = 11, 	FloorStep = 12, 	FloorTile = 13, 	FloorWater = 14, 	FloorMetal = 15, 	FloorWood = 16, 	DeadlyNormal = 50, 	DeadlyExplosion = 51, 	DeadlyToxic = 52, 	DeadlyAbyss = 53 };
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
public enum EntityType { 	Info_T = 0, 	Info_CT = 1, 	Info_VIP = 2, 	Info_Hostage = 3, 	Info_RescuePoint = 4, 	Info_BombSpot = 5, 	Info_EscapePoint = 6, 	Info_Animation = 8, 	Info_Storm = 9, 	Info_TileFX = 10, 	Info_NoBuying = 11, 	Info_NoWeapons = 12, 	Info_NoFOW = 13, 	Info_Quake = 14, 	Info_CTF_Flag = 15, 	Info_OldRender = 16, 	Info_Dom_Point = 17, 	Info_NoBuildings = 18, 	Info_BotNode = 19, 	Info_TeamGate = 20, 	Info_NoWeather = 80, 	Info_RadarIcon = 81, 	Evn_Item = 21, 	Evn_Sprite = 22, 	Evn_Sound = 23, 	Evn_Decal = 24, 	Evn_Breakable = 25, 	Evn_Explode = 26, 	Evn_Hurt = 27, 	Evn_Image = 28, 	Evn_Object= 29, 	Evn_Building = 30, 	Evn_NPC = 31, 	Evn_Room = 32, 	Evn_Light = 33, 	Evn_LightStripe = 34, 	Gen_Particles = 50, 	Gen_Sprites = 51, 	Gen_Weather = 52, 	Gen_FX = 53, 	Func_Teleport = 70, 	Func_DynWall = 71, 	Func_Message = 72, 	Func_GameAction = 73, 	Trigger_Start = 90, 	Trigger_Move = 91, 	Trigger_Hit = 92, 	Trigger_Use = 93, 	Trigger_Delay = 94, 	Trigger_Once = 95, 	Trigger_If = 96 };
Cs2dMapLib.IO 

Classes:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
Class: MapReader //It used to map reading Constructor: public MapReader(string path) //Opens the map reading along a predetermined path Methods: public string ReadString() //Read string public int ReadInt() //Read integer public byte ReadByte() //Read byte public string[] ReadStrings(int Count) //Read string array public int[] ReadInts(int Count) //Read integer array public byte[] ReadBytes(int Count) //Read byte array public void Close() //Ends the reading and closes the file Properties: private FileStream stream; //File stream private BinaryReader reader; //Binary reader
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
Class: MapWriter //Used to create and write to the data from the map file Constructor: public MapWriter(string path) //It creates a file for writing at a given path Methods: public void Write(string String) //Write string public void Write(int Int) //Write integer public void Write(byte Byte) //Write byte public void Write(string[] Strings) //Write string array public void Write(int[] Ints) //Write integer array public void Write(byte[] Bytes) //Write byte array public void Close() //Ends the writing and closes the file Properties: private FileStream stream; //File stream private BinaryWriter writer; //Binary writer
Example 1 

Open and save maps removing entity that fall under this
condition.
condition.
1
2
3
4
5
6
7
8
2
3
4
5
6
7
8
Map map = new Map("LoadPath"); List<Entity> RemEntity = new List<Entity>(); //Remove list entity foreach (Entity ent in map.Entites) 	if (ent.Type == EntityType.Evn_Sprite || (ent.Position.X > 50 && ent.Position.Y > 40 && ent.Position.Y < 60)) 		RemEntity.Add(ent); foreach (Entity ent in RemEntity) 	map.Entites.Remove(ent); map.Save("SavePath");
Example 2 

Creating and generating a new map.
Result:
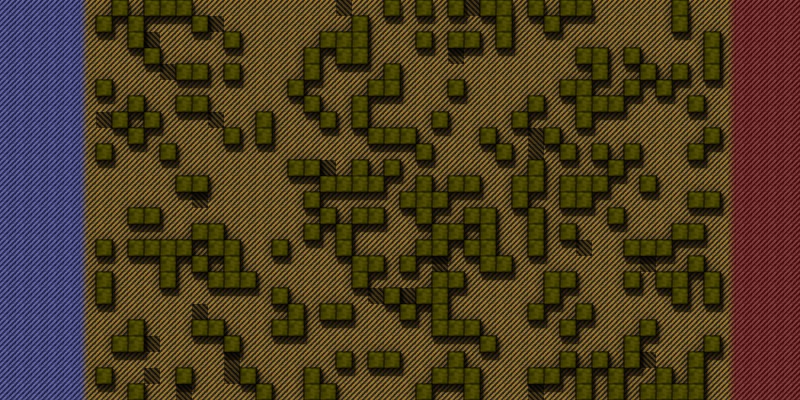
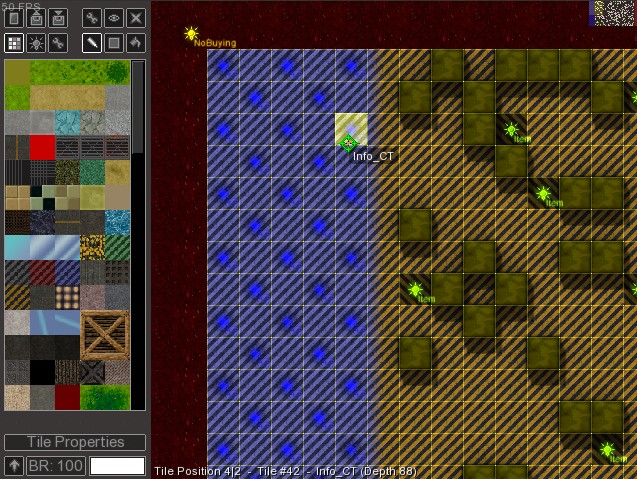
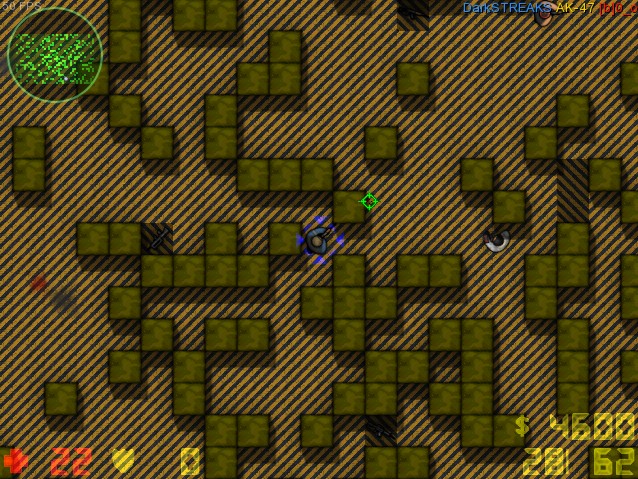
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
Map map = new Map(50, 25); map.AuthorId = 0; //USGN map.AuthorNick = "Example"; map.DaylightTime = 1250; //map.DaylightTime 1250 == 1(250) = 250. Min=1000; Max=1360; 0 = Default (225) map.BackName = "blood.jpg"; map.BackColor = new Cs2dMapLib.TColor(255, 0, 0); map.BackScroling = true; map.BackScrollSpeed = new XY(5, 5); map.TilesName = "cs2dnorm.bmp"; map.TSet = new TileSet("TileSetPath"); //For example, cs2dnorm.inf bool placeEnt = true; Random rand = new Random(); for (int x = 0; x < map.TMap.SizeX; x++) 	for(int y = 0; y < map.TMap.SizeY; y++) 	{ 		TileInfo t = map.TMap.Tiles[x, y]; 		if (x < 5) 		{ 			t.Tile = 42; 			if(placeEnt) { 				Entity ent = new Entity(EntityType.Info_CT, new XY(x, y)); 				ent.Ints[0] = 90; //Entity Properties. Rotate 				map.Entites.Add(ent); } 			placeEnt = !placeEnt; 		} 		else if (x > 45) 		{ 			t.Tile = 41; 			if (placeEnt) { 				Entity ent = new Entity(EntityType.Info_T, new XY(x, y)); 				ent.Ints[0] = -90; //Entity Properties. Rotate 				map.Entites.Add(ent); } placeEnt = !placeEnt; 		} 		else if (x == 5) 		{ 			t.Tile = 45; 			t.SetBlend(BlentType.LinearS, BlendRotate.r_270); 		} 		else if (x == 45) 		{ 			t.Tile = 45; 			t.SetBlend(BlentType.LinearS, BlendRotate.r_90); 		} 		else 		{ 			if (rand.Next(0, 4) == 0) 				t.Tile = 105; 			else { 				t.Tile = 45; 				if (rand.Next(0, 30) == 0) 				{ 					t.TRotation = Rotation.Left; 					t.TBrightness = Brightness.b_50; 					Entity ent = new Entity(EntityType.Evn_Item, new XY(x, y)); 					//Item Id 					switch (rand.Next(0, 6)) 					{ 						case 0: 							ent.Ints[0] = 30; 							break; 						case 1: 							ent.Ints[0] = 32; 							break; 						case 2: 							ent.Ints[0] = 38; 							break; 						case 3: 							ent.Ints[0] = 39; 							break; 						case 4: 							ent.Ints[0] = 11; 							break; 						case 5: 							ent.Ints[0] = 51; 							break; 					} 					ent.Ints[1] = 2; //Spawn: Every x Sec 					ent.Ints[2] = 5; //5 Sec 					map.Entites.Add(ent); 				} 			} 		} 	} 	map.Entites.Add(new Entity(EntityType.Info_NoBuying, new XY(-1, -1))); 	map.Save("D:\\Counter-Strike 2D\\maps\\TestMap.map");
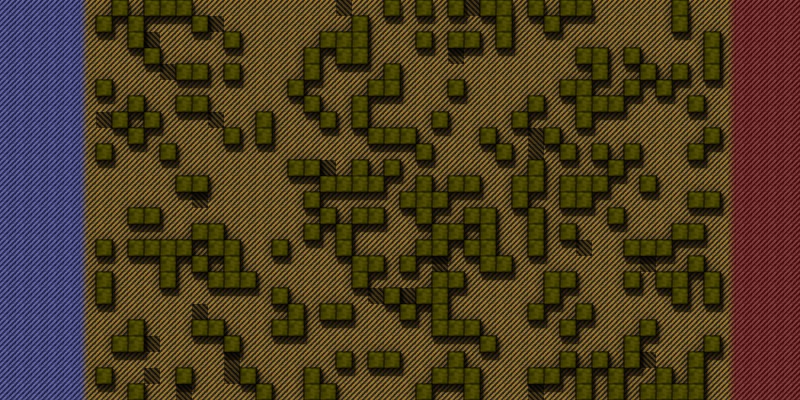
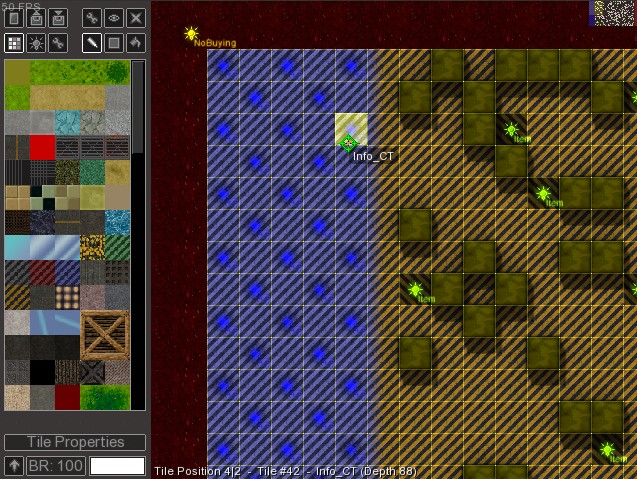
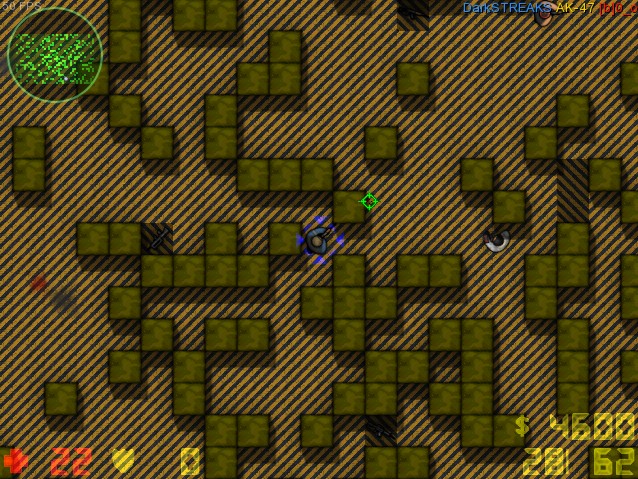


edited 28×, last 23.09.16 04:39:51 pm
Approved by Sparty
Download
15 kb, 308 Downloads